HPC submit nodes do not have enough memory to carry out JAVA VM, therefore we need to do:
SSH -Y TEST04
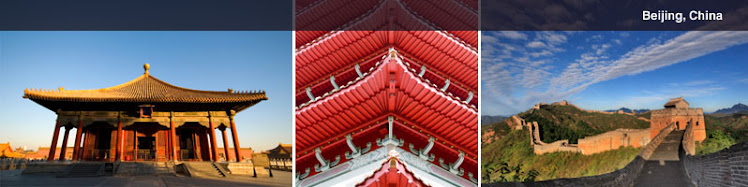
Thursday, December 29, 2011
Wednesday, July 20, 2011
Start MySql with launchd.plist
1. Create a mysql plist file on /Library/LaunchDaemons/com.mysql.mysqld.plis
2. Start MySql Server
sudo launchctl load -w /Library/LaunchDaemons/com.mysql.mysqld.plist
3. Stop MySql Server
sudo launchctl unload -w /Library/LaunchDaemons/com.mysql.mysqld.plist
How to uninstall Mysql in MacOS
Do this command first:
vim /etc/hostconfig and removed the line MYSQLCOM=-YES-
Then do the following lines:
sudo rm /usr/local/mysql
sudo rm -rf /usr/local/mysql*
sudo rm -rf /Library/StartupItems/MySQLCOM
sudo rm -rf /Library/PreferencePanes/My*
sudo rm -rf /Library/Receipts/mysql*
sudo rm -rf /Library/Receipts/MySQL*
sudo rm -rf /var/db/receipts/com.mysql.*
sudo rm /etc/my.cnf
rm -rf ~/Library/PreferencePanes/My*
Do not forget to remove the my.cnf file.
vim /etc/hostconfig and removed the line MYSQLCOM=-YES-
Then do the following lines:
sudo rm /usr/local/mysql
sudo rm -rf /usr/local/mysql*
sudo rm -rf /Library/StartupItems/MySQLCOM
sudo rm -rf /Library/PreferencePanes/My*
sudo rm -rf /Library/Receipts/mysql*
sudo rm -rf /Library/Receipts/MySQL*
sudo rm -rf /var/db/receipts/com.mysql.*
sudo rm /etc/my.cnf
rm -rf ~/Library/PreferencePanes/My*
Do not forget to remove the my.cnf file.
Tuesday, July 19, 2011
Monday, July 11, 2011
Spots
import java.awt.*;
public class Spots extends java.applet.Applet{
final int MaxSpots = 10;
int[] xPositions = new int[10];
int[] yPositions = new int[10];
int currentSpots = 0;
public void init() {
setBackground(Color.black);
}
public boolean mouseDown(Event evt, int x, int y){
if (currentSpots < MaxSpots){
addSpots(x,y);
}
else
{System.out.println("Too Many Spots.....\n");}
return true;
}
void addSpots(int x, int y){
xPositions[currentSpots] = x;
yPositions[currentSpots] = y;
currentSpots++;
repaint();
}
public void paint(Graphics g){
g.setColor(Color.blue);
for(int i=0; i < currentSpots; i++)
{
g.fillOval(xPositions[i] -10, yPositions[i] -10,20,20);
}
}
}
public class Spots extends java.applet.Applet{
final int MaxSpots = 10;
int[] xPositions = new int[10];
int[] yPositions = new int[10];
int currentSpots = 0;
public void init() {
setBackground(Color.black);
}
public boolean mouseDown(Event evt, int x, int y){
if (currentSpots < MaxSpots){
addSpots(x,y);
}
else
{System.out.println("Too Many Spots.....\n");}
return true;
}
void addSpots(int x, int y){
xPositions[currentSpots] = x;
yPositions[currentSpots] = y;
currentSpots++;
repaint();
}
public void paint(Graphics g){
g.setColor(Color.blue);
for(int i=0; i < currentSpots; i++)
{
g.fillOval(xPositions[i] -10, yPositions[i] -10,20,20);
}
}
}
Wednesday, June 29, 2011
Figure for Manuscript: Retinoic Acid Binding Site
Tuesday, June 14, 2011
Color Swirl in Both background and foreground
import java.awt.Font;
import java.awt.Graphics;
import java.util.Date;
import java.awt.Color;
public class ColorSwirl extends java.applet.Applet implements Runnable {
Font swirlFont = new Font("Arial", Font.BOLD, 24 );
Color[] colors = new Color[50];
Thread runner;
public void start() {
if (runner == null) {
runner = new Thread(this);
runner.start();
}
}
public void stop() {
if (runner != null) {
runner.stop();
runner = null;
}
}
public void update(Graphics g){
paint(g);
}
public void run() {
// Initialize the color array
float c = 0;
for (int i=0; i < colors.length; i++){
colors[i] = Color.getHSBColor(c, (float) 1.0, (float) 1.0);
c = c + 0.02f;
}
// Cycle through the colors
int j = 0;
while (true) {
setBackground(colors[49-j]);
setForeground(colors[j]);
repaint();
j++;
try { Thread.sleep(100); }
catch (InterruptedException e) {}
if (j == colors.length) j = 0;
}
}
public void paint (Graphics g) {
g.setFont(swirlFont);
g.drawString("I love my dear DaXiongXiong", 15, 50);
}
}
import java.awt.Graphics;
import java.util.Date;
import java.awt.Color;
public class ColorSwirl extends java.applet.Applet implements Runnable {
Font swirlFont = new Font("Arial", Font.BOLD, 24 );
Color[] colors = new Color[50];
Thread runner;
public void start() {
if (runner == null) {
runner = new Thread(this);
runner.start();
}
}
public void stop() {
if (runner != null) {
runner.stop();
runner = null;
}
}
public void update(Graphics g){
paint(g);
}
public void run() {
// Initialize the color array
float c = 0;
for (int i=0; i < colors.length; i++){
colors[i] = Color.getHSBColor(c, (float) 1.0, (float) 1.0);
c = c + 0.02f;
}
// Cycle through the colors
int j = 0;
while (true) {
setBackground(colors[49-j]);
setForeground(colors[j]);
repaint();
j++;
try { Thread.sleep(100); }
catch (InterruptedException e) {}
if (j == colors.length) j = 0;
}
}
public void paint (Graphics g) {
g.setFont(swirlFont);
g.drawString("I love my dear DaXiongXiong", 15, 50);
}
}
Tuesday, June 7, 2011
Monday, June 6, 2011
How to create 4 dimensional Vector
import java.awt.Point;
class FourDPoint extends Point {
/**
* @param args
*/
int threeDimension, fourDimension;
FourDPoint(int x, int y, int threeD, int fourD){
super(x,y);
this.threeDimension = threeD;
this.fourDimension = fourD;
}
public static void main(String[] args) {
// TODO Auto-generated method stub
FourDPoint fourDimPoint = new FourDPoint(5, 10, 120, 600);
System.out.println("The First Dimension is: " + fourDimPoint.x);
System.out.println("The Second Dimension is: " + fourDimPoint.y);
System.out.println("The Third Dimension is: " + fourDimPoint.threeDimension);
System.out.println("The Fourth Dimension is: " + fourDimPoint.fourDimension);
}
}
class FourDPoint extends Point {
/**
* @param args
*/
int threeDimension, fourDimension;
FourDPoint(int x, int y, int threeD, int fourD){
super(x,y);
this.threeDimension = threeD;
this.fourDimension = fourD;
}
public static void main(String[] args) {
// TODO Auto-generated method stub
FourDPoint fourDimPoint = new FourDPoint(5, 10, 120, 600);
System.out.println("The First Dimension is: " + fourDimPoint.x);
System.out.println("The Second Dimension is: " + fourDimPoint.y);
System.out.println("The Third Dimension is: " + fourDimPoint.threeDimension);
System.out.println("The Fourth Dimension is: " + fourDimPoint.fourDimension);
}
}
Thursday, June 2, 2011
java.util.StringTokenizer
import java.util.StringTokenizer;
class showToken {
/**
* @param args
*/
public static void main(String[] args) {
// TODO Auto-generated method stub
StringTokenizer stringOne;
String myBirthdayMonth, myBirthdayDate, myBirthdayYear;
String quoteOne = "01/22/2010/";
stringOne = new StringTokenizer(quoteOne, "/");
myBirthdayMonth = stringOne.nextToken();
myBirthdayDate = stringOne.nextToken();
myBirthdayYear = stringOne.nextToken();
System.out.println("My Birthday Month: " + myBirthdayMonth);
System.out.println("My Birthday Date: " + myBirthdayDate);
System.out.println("My Birthday Year: " + myBirthdayYear);
}
}
class showToken {
/**
* @param args
*/
public static void main(String[] args) {
// TODO Auto-generated method stub
StringTokenizer stringOne;
String myBirthdayMonth, myBirthdayDate, myBirthdayYear;
String quoteOne = "01/22/2010/";
stringOne = new StringTokenizer(quoteOne, "/");
myBirthdayMonth = stringOne.nextToken();
myBirthdayDate = stringOne.nextToken();
myBirthdayYear = stringOne.nextToken();
System.out.println("My Birthday Month: " + myBirthdayMonth);
System.out.println("My Birthday Date: " + myBirthdayDate);
System.out.println("My Birthday Year: " + myBirthdayYear);
}
}
Wednesday, June 1, 2011
How to determine if an element exists in a Perl array
#!/usr/bin/perl
my @testArray = qw(apple apple1 apple2 banana);
my $testElement1 = "apple1";
my $testElement2 = "banana";
my $Counter = 0; my %testHash = {};
%testHash = map { $_ => $Counter++} @testArray;
print "Test element 1 exists!\n" if (exists $testHash{$testElement1});
print "Test element 2 exists!\n" if (exists $testHash{$testElement2});
my @testArray = qw(apple apple1 apple2 banana);
my $testElement1 = "apple1";
my $testElement2 = "banana";
my $Counter = 0; my %testHash = {};
%testHash = map { $_ => $Counter++} @testArray;
print "Test element 1 exists!\n" if (exists $testHash{$testElement1});
print "Test element 2 exists!\n" if (exists $testHash{$testElement2});
Mysql Issue
Yesterday I installed Mysql on my laptop. After running mysqld_safe it always gave me the same error information:
wipa-43-181:~ ZHENGFU$ mysqladmin version
/usr/local/mysql/bin/mysqladmin: connect to server at 'localhost' failed
error: 'Can't connect to local MySQL server through socket '/tmp/mysql.sock' (2)'
Check that mysqld is running and that the socket: '/tmp/mysql.sock' exists!
Seems like the mysql server did not start so the socket file was not created. Does anyone know how to figure it out?
wipa-43-181:~ ZHENGFU$ mysqladmin version
/usr/local/mysql/bin/mysqladmin: connect to server at 'localhost' failed
error: 'Can't connect to local MySQL server through socket '/tmp/mysql.sock' (2)'
Check that mysqld is running and that the socket: '/tmp/mysql.sock' exists!
Seems like the mysql server did not start so the socket file was not created. Does anyone know how to figure it out?
Hello World! My First Blog!
public class HelloWord {
/**
* @param args
*/
public static void main(String[] args) {
// TODO Auto-generated method stub
System.out.println("Hello world! My First Blog!");
}
}
/**
* @param args
*/
public static void main(String[] args) {
// TODO Auto-generated method stub
System.out.println("Hello world! My First Blog!");
}
}
Subscribe to:
Posts (Atom)